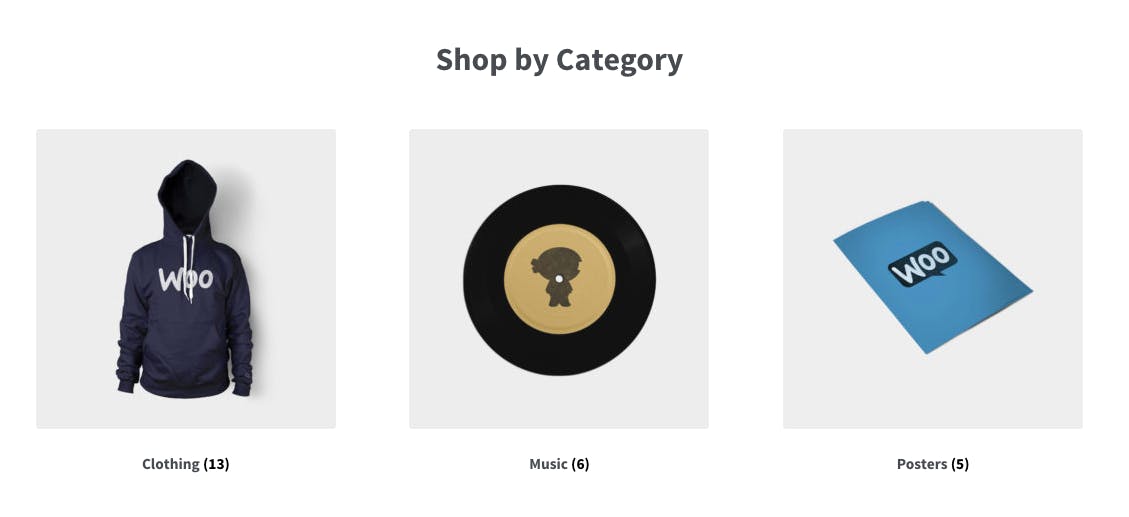
Show Random WooCommerce Products from a Category
A custom WooCommerce extension script, called via a shortcode, to show a list of random products from a category. This script was actually created for a client who uses the Avada theme. The Avada theme overrides most of the <a href="https://docs.woocommerce.com/document/woocommerce-shortcodes/" target="_blank" rel="noopener noreferrer">WooCommerce product display shortcodes </a>
with their own library so it makes certain parameters unavailable.
This script can be added to the theme's function.php file.
The Shortcode Function
Shortcode Example:
We want to display 4x products from the category "20" in 4x columns.
<pre class="wp-block-preformatted">[woocommerce-custom-category id="20" display="4" columns="4"]</pre>
First we would need to create a new shortcode hook that in return referance a callback function called: "woocommerce_custom_category_shortcode".
add_shortcode('woocommerce-custom-category', 'woocommerce_custom_category_shortcode')
Now we can create the function called.: "woocommerce_custom_category_shortcode".
/**
* Display a column list of random products from a selected category.
* @param category_id = Declare your category ID.
* @param display = Number of posts to display.
* @param columns = Define the number of columns to display.
*/
public function woocommerce_custom_category_shortcode( $atts ) {
//We collect the post object that contains all the posts gathered by Wordpress.
global $post;
//Declare default values for our shortcode.
$shortcode_att = shortcode_atts( array(
'category_id' => '',
'display' => '4',
'columns' => '4',
), $atts );
//extract the attributes and place them in variables.:
$display = $shortcode_att['display'];
$category_ids = explode(',', $shortcode_att['category_id'] );
$columns = $shortcode_att['columns'];
$args = array(
'post_type' => 'product', // WooCommerce Post Type
'tax_query' => array(
array(
'taxonomy' => 'product_cat', // WooCommerce Taxonomy
'field' => 'id', // Focus on ID field.
'terms' => $category_ids, //We add the Category ID to the DB arguments array
'operator'=> 'IN' //Or 'AND' or 'NOT IN'
)),
'posts_per_page' => $display,// Number of products to display
'ignore_sticky_posts' => 1,
'orderby' => 'rand',// Randomize the selection.
);
// Get the results from the database and return it as a object.
$query_result = new WP_Query($args);
if ( $query_result->have_posts() ) { ?>
<?php ob_start(); ?>
<div class="woocommerce columns-<?php echo $columns; ?>">
<ul class="products clearfix products-<?php echo $display; ?>">
<!-- loop start -->
<?php while( $query_result->have_posts() ) : $query_result->the_post(); ?>
<?php $product = new WC_Product( $post->ID ); ?>
<?php // Get the the current's post categories and only filter the child-categories for the class values.
$cargs = array( 'parent' => 0 );
$cat_terms = wp_get_post_terms( $post->ID, 'product_cat', $cargs );
$recorded_terms = '';
foreach ($cat_terms as $cat_term ) {
$recorded_terms .= ' product_cat-'. $cat_term->slug;
}
?>
<!-- product image -->
<li class="post-<?php echo get_the_ID(); ?> product type-product <?php echo get_post_status( $post->ID ); ?> <?php echo ( has_post_thumbnail( $post->ID ) ) ? 'has-post-thumbnail' : ''; ?> <?php echo 'product-type-' . wc_get_product()->product_type; ?><?php echo $recorded_terms; ?>">
<a class="product-images" href="<?php the_permalink(); ?>">
<!-- <span class="featured-image"><?php //the_post_thumbnail('medium'); ?></span> -->
<span class="featured-image"><?php echo woocommerce_get_product_thumbnail( $size = 'shop_catalog', $deprecated1 = 0, $deprecated2 = 0 ); ?></span>
</a>
<!-- product details -->
<div class="product-details">
<div class="product-details-container">
<div class="productCatIcon" style="display: block;"></div>
<div class="productTagIcon" style="display: block;"></div>
<h3 class="product-title">
<a href="<?php the_permalink(); ?>"><?php the_title(); ?></a>
</h3>
<div class="clearfix">
<span class="price">
<span class="woocommerce-Price-amount amount">
<?php if ( ! empty( $product->get_price() )) { ?>
<?php echo $product->get_price_html(); ?>
</span>
</span>
<?php } ?>
</div>
</div>
</div>
<!-- product button -->
<div class="product-buttons">
<div class="product-buttons-container clearfix">
<a rel="nofollow" href="/?add-to-cart=<?php echo $post->ID; ?>" data-quantity="1" data-product_id="<?php echo $post->ID; ?>" data-product_sku="<?php echo $product->get_sku(); ?>" class="button product_type_<?php echo 'product-type-' . wc_get_product()->product_type; ?> add_to_cart_button ajax_add_to_cart">Add to basket</a>
<a href="<?php the_permalink(); ?>" class="show_details_button">Details</a>
</div>
</div>
</li>
<?php endwhile; ?>
<!-- loop end -->
</ul>
</div> <!-- end columns -->
<?php return ob_get_clean(); ?>
<?php wp_reset_query(); ?>
<?php } ?>
<?php }